Introduction
I’m excited to announce the first public release of Woo Yearly Credits—a WordPress/WooCommerce plugin that provides a yearly store credit to your site’s users and handles everything from partial usage to full store-credit gateway functionality. You can now find, download, or contribute to the code on my GitHub repository!
The Issue at Hand
Woo Yearly Credits gives each of your users (e.g., employees, members, etc.) a fixed annual credit allowance (like $400) to spend on your WooCommerce store. Every year, the credits automatically reset on a specific month/day, and any unused credits don’t roll over. The plugin also includes:
- A full store-credit gateway (no tax charged if chosen).
- An optional partial-credit flow (users generate a one-time coupon for the amount of credit they have).
- Admin tools to reset credits for all or individual users.
- A daily cron cleanup to remove stale coupons.
- A read-only display of each user’s credits in their WP Admin user profile.
Key Highlights (with Code Snippets)
Below are some of the cool features inside the codebase. Everything is meticulously separated into logical classes under an includes/
directory, so it’s easy to browse and maintain.
Automatic Yearly Reset Logic
Thanks to a daily cron job, Woo Yearly Credits checks the current date against your configured reset month/day. On the designated date, all user credits are reset to the default amount. This is found in class-woo-yearly-credits-cron.php. Here’s a snippet:
public function handle_daily_cron() {
$month = get_option( 'wyc_yearly_credit_reset_month', '1' );
$day = get_option( 'wyc_yearly_credit_reset_day', '1' );
$target_md = sprintf( '%02d-%02d', intval( $month ), intval( $day ) );
$today_md = date( 'm-d', current_time( 'timestamp' ) );
if ( $today_md === $target_md ) {
$amount = get_option( 'wyc_yearly_credit_amount', 400 );
self::reset_all_user_credits( $amount );
}
self::cleanup_stale_coupons();
}
In short, once a day, it checks if today is “reset day.” If so, all users get their credits set back to the configured amount!
Partial Usage via One-Time Coupon
One of the unique features is partial usage. If a user’s credit can’t fully cover the order total, they can generate a single-use coupon for just the amount of credit they have. This ensures the partial credit is still deducted properly at checkout. The code in class-woo-yearly-credits-partial.php handles it:
if ( $balance > 0 && $cart_total > 0 && $balance < $cart_total ) {
$coupon_amount = min( $balance, $cart_total );
$coupon_code = 'credits-' . substr( md5( uniqid( '', true ) ), 0, 10 );
$coupon_id = wp_insert_post( array(
'post_title' => $coupon_code,
'post_content' => __( 'Auto-generated one-time store-credit coupon.', 'woo-yearly-credits' ),
'post_status' => 'publish',
'post_author' => 1,
'post_type' => 'shop_coupon',
) );
// Then sets meta for discount, usage limit = 1, etc.
// Finally, WC()->cart->add_discount( $coupon_code );
}
We generate a random coupon code, create a new shop_coupon
post in WordPress, limit usage to 1
, and apply it directly to the cart. Once the user checks out, the code automatically deducts that amount from their credit balance.
Full Store Credits Gateway (No Tax!)
If the user’s credit covers the entire order, they see a Store Credits payment gateway that can fully handle the purchase—and it removes all tax calculations from the order. If they click “Store Credits” at checkout, the plugin zeros out the cart’s tax totals. The code in class-woo-yearly-credits-payment-gateway.php handles it:
add_action( 'woocommerce_calculate_totals', array( $this, 'remove_taxes_if_store_credits_gateway' ), 1000 );
public function remove_taxes_if_store_credits_gateway( $cart ) {
$chosen_method = WC()->session ? WC()->session->get( 'chosen_payment_method' ) : '';
$posted_method = isset( $_POST['payment_method'] ) ? sanitize_text_field( $_POST['payment_method'] ) : '';
if ( $chosen_method === $this->id || $posted_method === $this->id ) {
// Zero out tax totals
$cart->set_tax_total( 0 );
$cart->set_shipping_tax_total( 0 );
$cart->set_taxes( array() );
$cart->set_shipping_taxes( array() );
// Also ensure each line item tax is zero
foreach ( $cart->get_cart() as $cart_item_key => $cart_item ) {
$cart->cart_contents[ $cart_item_key ]['line_tax'] = 0;
$cart->cart_contents[ $cart_item_key ]['line_subtotal_tax'] = 0;
}
}
}
Tax-free if you pay 100% via store credits—very handy for businesses wanting to avoid additional complexities for employees or specific user groups.
Easy Admin Interface & Tools
We put everything under WooCommerce → Yearly Credits in the WP Admin. From here, you can:
- Set the default yearly credit amount.
- Choose your reset month/day.
- Toggle partial usage.
- Pick colors for your partial credit button.
- Reset credits for all users or a single user via email/user ID.
User Profile pages also show a read-only view of the user’s current credit:
Admin Page Screenshot
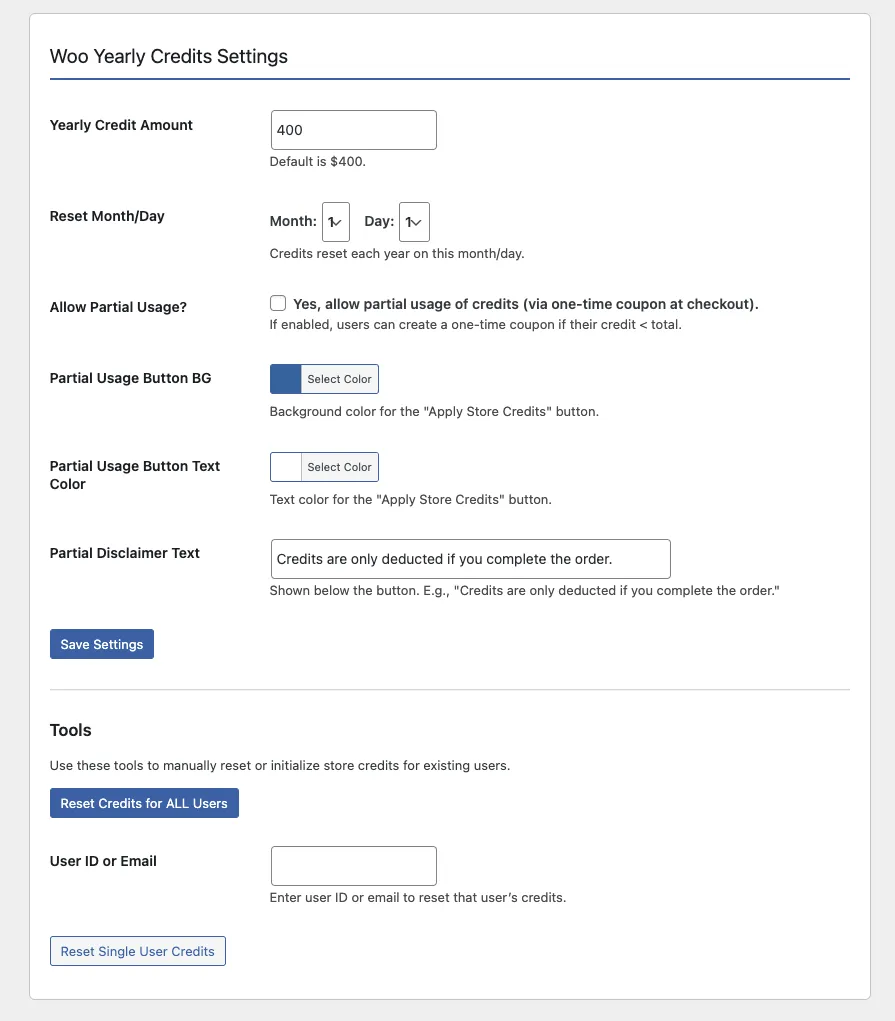
Grab It Now on GitHub!
This is my v1.0.0 public release. I’m excited to see how store owners and users start using it:
- GitHub Repository:
https://github.com/altenderfer/woo-yearly-credits
Feel free to star the repo, open issues, or submit pull requests. I’d love your feedback on how to make Woo Yearly Credits even better.
Installation & Setup
- Clone or download the repo into
wp-content/plugins/woo-yearly-credits
. - Activate the plugin in Plugins → Installed Plugins.
- Configure via WooCommerce → Yearly Credits.
- Optionally check the partial usage box if you want to enable one-time coupons for smaller balances.
- Done!
What’s Next?
We’ll continue to refine the plugin based on community feedback. Potential updates include:
- Additional reporting to see how many credits are used/left across all employees.
- More advanced usage restrictions or role-based conditions.
- Import/export for custom credit amounts.
Stay tuned, and I look forward to hearing how you put Woo Yearly Credits to use in your WooCommerce store!